New JavaScript Set Methods
Unlock the power of modern JavaScript with the latest Set methods! In this guide, we'll explore the newest Set methods that enhance your coding efficiency and simplify data management. Discover how to use these methods to handle collections of unique values with ease, improve performance, and write cleaner code. Whether you're a seasoned developer or just getting started, mastering these methods will give you a strong edge in your JavaScript projects.
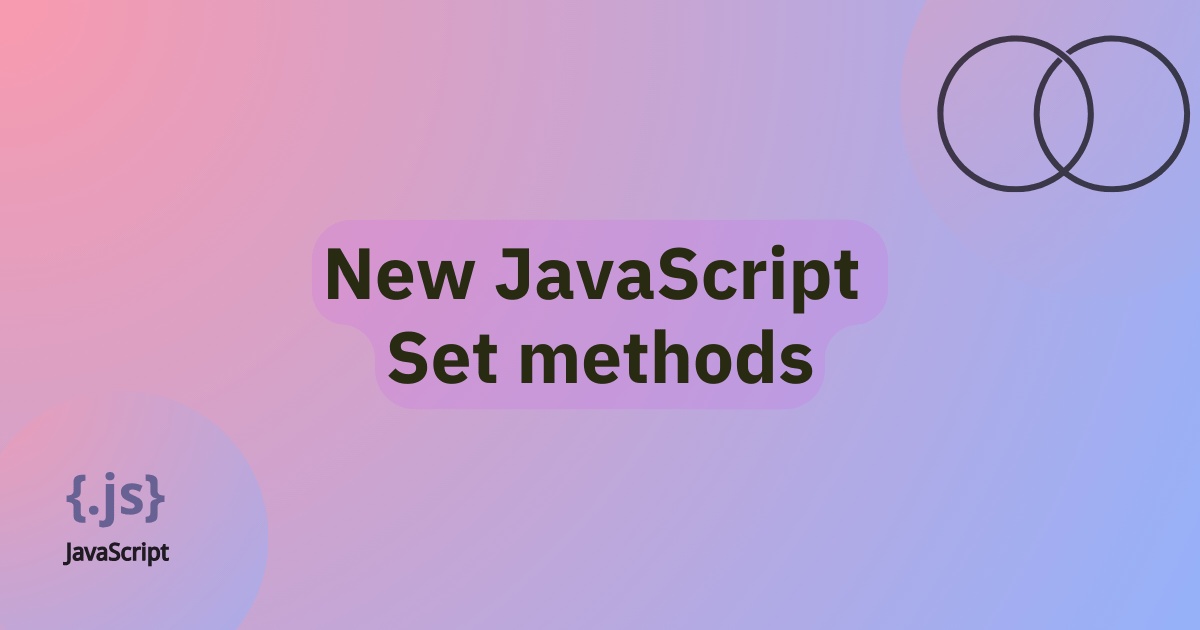
JavaScript, a dynamic and versatile language, continues to evolve with each new version, introducing enhanced functionalities and more efficient ways to handle data. Among the notable additions in recent updates are new methods for the Set
object. Sets in JavaScript provide a collection of unique values and are ideal for scenarios where you need to ensure that no duplicates are present. With the latest updates, JavaScript has introduced several new methods to extend the functionality of Sets, making them even more powerful and flexible. This comprehensive guide explores the new JavaScript Set methods, their uses, and how they can enhance your development experience.
Introduction to JavaScript Sets
Before diving into the new methods, it's important to understand the basic concept of JavaScript Sets. A Set is a built-in data structure that stores unique values of any type. Unlike arrays, Sets do not allow duplicate elements, and they offer fast access to individual items. Sets are particularly useful when you need to maintain a collection of items with no repetition.
The New Methods Added to Sets
Recent updates to JavaScript have introduced new methods for Sets that enhance their capabilities. These methods offer improved functionality and make it easier to perform complex operations. Let’s explore each of these new methods in detail.
Set.prototype.addAll
The addAll
method is a new addition that simplifies the process of adding multiple values to a Set. Before this method, adding multiple values required a loop or a combination of multiple add
calls. With addAll
, you can add an array or another iterable of values in a single operation.
Here’s how addAll
works:
mySet.addAll([1, 2, 3, 4]);
console.log(mySet); // Set { 1, 2, 3, 4 }
let mySet = new Set(); mySet.addAll([1, 2, 3, 4]); console.log(mySet); // Set { 1, 2, 3, 4 }
This method makes it more convenient to initialize a Set with multiple values or to extend an existing Set with additional items.
Set.prototype.deleteAll
The deleteAll
method allows you to remove multiple values from a Set at once. Previously, if you needed to remove several items, you had to call delete
for each value individually. With deleteAll
, you can streamline this process by passing an array or iterable of values to be removed.
Example usage of deleteAll
:
mySet.deleteAll([2, 4]);
console.log(mySet); // Set { 1, 3 }
let mySet = new Set([1, 2, 3, 4]); mySet.deleteAll([2, 4]); console.log(mySet); // Set { 1, 3 }
This method is particularly useful when you need to perform bulk deletions from a Set, improving code readability and efficiency.
Set.prototype.clearAll
The clearAll
method provides a more controlled approach to clearing items from a Set. While the clear
method removes all elements from a Set, clearAll
allows you to specify which items to remove. This method takes an array or iterable of values and removes only those items from the Set.
Here’s an example of clearAll
in action:
mySet.clearAll([2, 4]);
console.log(mySet); // Set { 1, 3, 5 }
let mySet = new Set([1, 2, 3, 4, 5]); mySet.clearAll([2, 4]); console.log(mySet); // Set { 1, 3, 5 }
By allowing selective clearing, clearAll
helps manage Set data more precisely.
Set.prototype.union
The union
method allows you to create a new Set that is the union of the current Set and another Set or iterable. This method combines all unique values from both Sets into a new Set, effectively merging them while preserving uniqueness.
Example usage of union
:
let setB = new Set([3, 4, 5]);
let setC = setA.union(setB);
console.log(setC); // Set { 1, 2, 3, 4, 5 }
let setA = new Set([1, 2, 3]); let setB = new Set([3, 4, 5]); let setC = setA.union(setB); console.log(setC); // Set { 1, 2, 3, 4, 5 }
This method is useful when you need to combine Sets or merge data from different sources.
Set.prototype.intersection
The intersection
method creates a new Set containing only the values that are present in both the current Set and another Set or iterable. This method is valuable when you want to find common elements between Sets.
Example of using intersection
:
let setB = new Set([2, 3, 4]);
let setC = setA.intersection(setB);
console.log(setC); // Set { 2, 3 }
let setA = new Set([1, 2, 3]); let setB = new Set([2, 3, 4]); let setC = setA.intersection(setB); console.log(setC); // Set { 2, 3 }
By using intersection
, you can efficiently determine shared values between different Sets.
Set.prototype.difference
The difference
method returns a new Set containing values that are in the current Set but not in another Set or iterable. This method helps identify unique items in one Set relative to another.
Example of difference
in action:
let setB = new Set([2, 3, 4]);
let setC = setA.difference(setB);
console.log(setC); // Set { 1 }
let setA = new Set([1, 2, 3]); let setB = new Set([2, 3, 4]); let setC = setA.difference(setB); console.log(setC); // Set { 1 }
The difference
method is particularly useful for finding items that are exclusive to one Set.
Set.prototype.symmetricDifference
The symmetricDifference
method returns a new Set containing values that are in either the current Set or another Set but not in both. This method provides a way to identify items that are unique to each Set.
Here’s an example:
let setB = new Set([2, 3, 4]);
let setC = setA.symmetricDifference(setB);
console.log(setC); // Set { 1, 4 }
let setA = new Set([1, 2, 3]); let setB = new Set([2, 3, 4]); let setC = setA.symmetricDifference(setB); console.log(setC); // Set { 1, 4 }
Using symmetricDifference
, you can find values that differ between two Sets.
Set.prototype.isSubset
The isSubset
method checks if the current Set is a subset of another Set or iterable. This method returns a boolean value indicating whether all elements of the current Set are also present in the specified Set.
Example usage:
let setB = new Set([1, 2, 3]);
console.log(setA.isSubset(setB)); // true
let setA = new Set([1, 2]); let setB = new Set([1, 2, 3]); console.log(setA.isSubset(setB)); // true
This method is helpful for determining if one Set is contained within another.
Set.prototype.isSuperset
The isSuperset
method is the reverse of isSubset
. It checks if the current Set contains all elements of another Set or iterable. This method returns a boolean value indicating whether the current Set is a superset of the specified Set.
Example of using isSuperset
:
let setB = new Set([1, 2]);
console.log(setA.isSuperset(setB)); // true
let setA = new Set([1, 2, 3]); let setB = new Set([1, 2]); console.log(setA.isSuperset(setB)); // true
The isSuperset
method helps verify if a Set includes all elements of another Set.
Set.prototype.equals
The equals
method compares the current Set with another Set or iterable to determine if they are equal. This method returns a boolean value indicating whether both Sets contain exactly the same elements.
Example usage of equals
:
let setB = new Set([1, 2, 3]);
let setC = new Set([3, 2, 1]);
console.log(setA.equals(setB)); // true
console.log(setA.equals(setC)); // true
let setA = new Set([1, 2, 3]); let setB = new Set([1, 2, 3]); let setC = new Set([3, 2, 1]); console.log(setA.equals(setB)); // true console.log(setA.equals(setC)); // true
By using equals
, you can easily compare Sets for equality.
Set.prototype.toArray
The toArray
method converts a Set into an array. This method is useful when you need to work with Set data in an array format, for example, to perform array-specific operations or pass the data to functions that require arrays.
Here’s how toArray
works:
let array = mySet.toArray();
console.log(array); // [1, 2, 3]
let mySet = new Set([1, 2, 3]); let array = mySet.toArray(); console.log(array); // [1, 2, 3]
Using toArray
, you can seamlessly transform Set data into an array.
Set.prototype.fromArray
The fromArray
method allows you to create a new Set from an array or iterable. This method simplifies the process of initializing a Set with array data, avoiding the need for manual loops or the add
method.
Example of fromArray
:
let mySet = Set.fromArray(array);
console.log(mySet); // Set { 1, 2, 3 }
let array = [1, 2, 3]; let mySet = Set.fromArray(array); console.log(mySet); // Set { 1, 2, 3 }
With fromArray
, you can quickly convert array data into a Set.
Best Practices for Using New Set Methods
To make the most out of the new Set methods, consider the following best practices:
Understand the Use Cases
Choose the appropriate method based on your specific use case. For example, use union
to merge Sets, intersection
to find common elements, and difference
to identify unique items.
Leverage Method Chaining
Many of the new Set methods return a new Set, allowing for method chaining. Utilize this feature to perform multiple operations in a concise manner.
Optimize Performance
When dealing with large Sets, be mindful of performance implications. Methods that involve iteration or comparison may impact performance, so optimize your code as needed.
Combine with Other Data Structures
Consider combining Sets with other data structures like arrays or maps to achieve more complex data manipulation and management tasks.
What's Your Reaction?
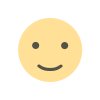
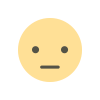
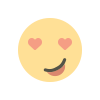
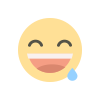
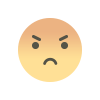
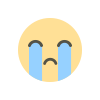
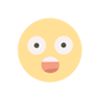